Double Range Slider with Min-Max value using HTML, CSS, & JavaScript
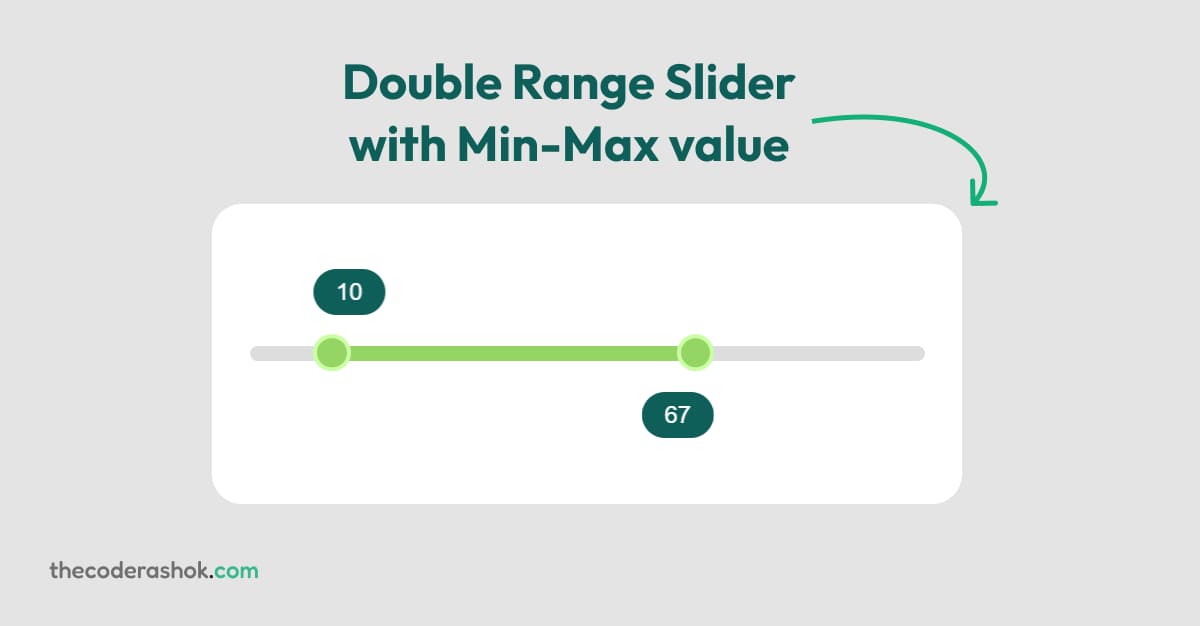
Double Range Slider with Min-Max value using HTML, CSS, & JavaScript
Introduction
Hello coders! We are talking about Double Range Slider | Double Range Slider with Min-Max value and today In this tutorial, you will learn to make Double Range Slider with Min-Max value using HTML, CSS, & JavaScript.
We will make an interactive design of this Double Range Slider with Min-Max value in floating bubbles at the top of its thumbs design, using HTML, CSS, and JavaScript.
Preview of the project
Project author | Ashok(Website owner) |
Built with | HTML, CSS, JavaScript |
Responsive | No |
Dependencies | - |
Compatible browsers | Chrome, Edge, Firefox, Opera, Safari |
Demo | Codepen preview here |
Steps to create Double Range Slider with Min-Max value using HTML, CSS, & JavaScript
Mainly we will go through 3 steps to create this Double Range Slider with Min-Max value.
Step 1: Create the basic structure HTML
Here I have structured this HTML of Double Range Slider with Min-Max value.
DIV with class double_range_slider_box
is our main box, inside this the div with class double_range_slider
is our range slider container in which I have added a span with class range_track
, and it will be our range track.
Also, I have created 2 div
with classes minvalue & maxvalue these will be our min-value bubble and max-value bubble, which will float at near the double range slider thumbs.
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta http-equiv="X-UA-Compatible" content="IE=edge" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="style.css" /> <title>Double Range Slider with Min-Max value</title> </head> <body> <div class="double_range_slider_box"> <div class="double_range_slider"> <span class="range_track" id="range_track"></span> <input type="range" class="min" min="0" max="100" value="0" step="0" /> <input type="range" class="max" min="0" max="100" value="20" step="0" /> <div class="minvalue"></div> <div class="maxvalue"></div> </div> </div> </body> <script src="main.js"></script> </html>
If you have noticed that I have added 2 <input type="range" >
tags inside the div whose class name is double_range_slider
.
<input type="range" class="min" min="0" max="100" value="0" step="0">
<input type="range" class="max" min="0" max="100" value="20" step="0">
Look at these input
tags type ranges, here which class name is min
that is for double range slider min-value and second which class name is max
that is for max-value.
By default I have set the first input value value="0"
and that second input value value="20"
these are the initial/default value before the user interacts. See the example below.
Read also: CSS Gradient Border Glowing Animation Hover Effect
Step 2: Create design using CSS
In the CSS part I have created some styles, like background-color
, transition
, width
, height
, margin
, padding
, etc.
css* { margin: 0; padding: 0; box-sizing: border-box; font-family: sans-serif; } body { position: relative; width: 100%; height: 100vh; display: flex; justify-content: center; align-items: center; background: rgb(228, 228, 228); } .double_range_slider_box { position: relative; width: 500px; height: 200px; background: white; display: flex; justify-content: center; align-items: center; border-radius: 20px; } .double_range_slider { width: 90%; height: 10px; position: relative; background-color: #dddddd; border-radius: 20px; } .range_track { height: 100%; position: absolute; border-radius: 20px; background-color: #95d564; } .minvalue { position: absolute; padding: 6px 15px; background: #0e5f59; border-radius: 1rem; color: white; bottom: 0; transform: translate(0, -100%); left: 0; font-size: 1rem; transition: left 0.3s cubic-bezier(0.165, 0.84, 0.44, 1); will-change: left, transform; } .maxvalue { position: absolute; padding: 6px 15px; background: #0e5f59; border-radius: 1rem; color: white; top: 0; transform: translate(0, 100%); right: 0; font-size: 1rem; transition: right 0.3s cubic-bezier(0.165, 0.84, 0.44, 1); will-change: right, transform; }
Remove the default style of <input type="range">
by using the below CSS code. Here you have noticed that, I have used here ::-webkit-slider-thumb
CSS pseudo-element & ::-moz-range-thumb
CSS pseudo-element to change the Input “thumb” style across all the browser.
::-webkit-slider-thumb | Chrome, Safari, Opera, Microsoft Edge, etc |
::-moz-range-thumb | Firefox |
cssinput { position: absolute; width: 100%; height: 5px; background: none; pointer-events: none; -webkit-appearance: none; -moz-appearance: none; top: 50%; transform: translateY(-50%); } input::-webkit-slider-thumb { height: 25px; width: 25px; border-radius: 50%; border: 3px solid #cbffa3; background-color: #95d564; pointer-events: auto; -webkit-appearance: none; cursor: pointer; margin-bottom: 1px; } input::-moz-range-thumb { height: 18px; width: 18px; border-radius: 50%; border: 3px solid #cbffa3; background-color: #95d564; pointer-events: auto; -moz-appearance: none; cursor: pointer; margin-top: 30%; }
Read also: Awesome Slider Animation using HTML, CSS, and JavaScript
Step 3: Add functionality to this Double Range Slider using JavaScript
NOTE! You must have little knowledge about JavaScript to understand and use this JavaScript code of Double Range Slider project.
In the JavaScript part I have created some variables to use these in functions.
javascriptlet minRangeValueGap = 6; const range = document.getElementById("range_track"); const minval = document.querySelector(".minvalue"); const maxval = document.querySelector(".maxvalue"); const rangeInput = document.querySelectorAll("input"); let minRange, maxRange, minPercentage, maxPercentage;
minRangeFill()
function is for fill the range track, when the user drag on “left-thumb” of double range slidermaxRangeFill()
function is for fill the range track, when user drag on “right-thumb”.MinVlaueBubbleStyle()
function is for Left bubble moving animation when user drag on “left-thumb”.MaxVlaueBubbleStyle()
function is for Right bubble moving animation when user drag on “right-thumb”.setMinValueOutput()
function for display range minimum value in the left bubble.setMaxValueOutput()
function for display range maximum value in the right bubble.
javascriptconst minRangeFill = () => { range.style.left = (rangeInput[0].value / rangeInput[0].max) * 100 + "%"; }; const maxRangeFill = () => { range.style.right = 100 - (rangeInput[1].value / rangeInput[1].max) * 100 + "%"; }; const MinVlaueBubbleStyle = () => { minPercentage = (minRange / rangeInput[0].max) * 100; minval.style.left = minPercentage + "%"; minval.style.transform = `translate(-${minPercentage / 2}%, -100%)`; }; const MaxVlaueBubbleStyle = () => { maxPercentage = 100 - (maxRange / rangeInput[1].max) * 100; maxval.style.right = maxPercentage + "%"; maxval.style.transform = `translate(${maxPercentage / 2}%, 100%)`; }; const setMinValueOutput = () => { minRange = parseInt(rangeInput[0].value); minval.innerHTML = rangeInput[0].value; }; const setMaxValueOutput = () => { maxRange = parseInt(rangeInput[1].value); maxval.innerHTML = rangeInput[1].value; };
Here I have return all the functions here.
Then I created a forEach()
function to rangeInput
variable and created a second addEventListener()
function to its child input elements the event is called “input”, this function is for when the user inputs the range or drags the range thumbs then run all the functions which we have previously made.
javascriptsetMinValueOutput() setMaxValueOutput() minRangeFill() maxRangeFill() MinVlaueBubbleStyle() MaxVlaueBubbleStyle() rangeInput.forEach((input) => { input.addEventListener("input", (e) => { setMinValueOutput(); setMaxValueOutput(); minRangeFill(); maxRangeFill(); MinVlaueBubbleStyle(); MaxVlaueBubbleStyle(); if (maxRange - minRange < minRangeValueGap) { if (e.target.className === "min") { rangeInput[0].value = maxRange - minRangeValueGap; setMinValueOutput(); minRangeFill(); MinVlaueBubbleStyle(); e.target.style.zIndex = "2" } else { rangeInput[1].value = minRange + minRangeValueGap; e.target.style.zIndex = "2" setMaxValueOutput(); maxRangeFill(); MaxVlaueBubbleStyle(); } } }); });
If you noticed that, I have created a condition in the if-else
statement is maxRange - minRange < minRangeValueGap
this is for when the user interacts with the double range slider and if the 2 thumbs get too close, the range will stop.
As example in this image:
Copy all the JavaScript code into you main.js
file.
Conclusion
This is the blog about Double Range Slider with Min-Max value using HTML, CSS, & JavaScript. Hope you like this blog and learned something new. If you have any doubt and questions about this project let me know in comments and ping me on Instagram.