CSS Gradient Border Glowing Animation Hover Effect
In this post, we will implement an interactive CSS gradient border glowing animation & effect of the cards on hover. Like, when the user hovers their mouse cursor over the card, they will see a glowing effect that goes out from the borders of the card.
The best part is that the glow effect will follow the mouse cursor's movement, which will be a great user experience.
Let's create a Gradient Border Glowing Animation Hover Effect using HTML, CSS, and JavaScript with step-by-step explanations.
How to create a Gradient Border Glowing Animation Hover Effect using HTML, CSS, and JavaScript
So, to build this project we will use HTML, CSS, and JavaScript,
- HTML: will be used to structure the layout.
- CSS: will be used to style the layout.
- JavaScript: to enable the functionality (track the movement of the mouse cursor over the cards and run the animation accordingly by updating the CSS variables, etc.)
Prerequisites
Before we dive into the implementation of Gradient Border Glowing Animation, make sure you have a basic knowledge of HTML, CSS, and JavaScript.
So, let's build this Gradient Border Glowing Animation project,
Preview of CSS Gradient Border Glowing Animation Hover Effect
Structure the layout using HTML
First of all links to our CSS stylesheet named "styles.css" to the HTML document.
Here, I have created 3 times, <div>
elements with the class "card" representing a card element with a title (<h1>
) and a description (<p>
). Additionally, cards contain another child <div>
element with the class "glow", which will be the container for the glowing border effect.
Then after the <body>
tag links to our JavaScript file.
html<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="styles.css" /> <title>Card Border Glow</title> </head> <body> <div class="card"> <div class="glow"></div> <h1>01</h1> <p>Move your mouse cursor over the card.</p> </div> <div class="card"> <div class="glow"></div> <h1>02</h1> <p>Move your mouse cursor over the card.</p> </div> <div class="card"> <div class="glow"></div> <h1>03</h1> <p>Move your mouse cursor over the card.</p> </div> </body> <script src="script.js"></script> </html>
Styling with CSS
Make sure you have linked your CSS file to the HTML document.
In CSS first of all I have imported fonts from Google Fonts . Then reset the default margin, padding and box-sizing properties.
Here, as you can see, I have created the CSS variable called --gradient
defines a conic gradient with multiple colour stops, which will be used with the background
property as gradient border & glow later in the code.
Then applied styles to the body, (<h1>
) and description (<p>
) elements.
css@import url("https://fonts.googleapis.com/css2?family=Inter:wght@500;600&family=Poppins:wght@400;500&display=swap"); * { margin: 0; padding: 0; box-sizing: border-box; font-family: "Poppins", sans-serif; } :root { --gradient: conic-gradient( from 90deg at 50% 50%, rgb(251, 55, 60), rgba(252, 114, 28, 1), rgba(255, 220, 0, 1), rgba(27, 206, 255, 1), rgba(42, 107, 255, 1), rgba(217, 41, 255, 1), rgba(255, 10, 92, 1) ); } body { display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; background-color: #0f0f0f; } h1 { font-size: 65px; color: rgb(71, 71, 71); text-align: center; font-weight: 600; } p { font-size: 20px; color: rgb(174, 174, 174); font-weight: 600; } .card { --start: 0; position: relative; display: flex; flex-direction: column; justify-content: center; align-items: flex-start; width: 280px; height: 350px; margin: 10px; padding: 10px 40px; background-color: #040404; border-radius: 14px; transition: border-color 0.3s ease-in-out; } .card::before { position: absolute; content: ""; width: 100%; height: 100%; left: 50%; top: 50%; transform: translate(-50%, -50%); border-radius: 14px; border: 2px solid transparent; background: var(--gradient); background-attachment: fixed; mask: linear-gradient(#0000, #0000), conic-gradient(from calc(( var(--start) - (20 * 1.1) ) * 1deg), #ffffff1f 0deg, white, #ffffff00 100deg); mask-composite: intersect; mask-clip: padding-box, border-box; opacity: 0; transition: 0.5s ease; }
How to create gradient border in CSS
In this project, the main part is to add a Gradient border to .card
elements using CSS. We will use conic-gradient()
CSS function and mask property to add a Gradient border to .card
elements.
So, first of all, I have added some styles to the .card
elements, like display to flex, flex-direction to the column, content alignments, width, height, margin, padding, border-radius, transition, etc.
You can notice here I have created a --start
custom property (CSS variable), defined to control the starting angle of the conic gradient. Its initial value is ‘0’, which will change dynamically through JavaScript.
Then I added styles to the card’s Pseudo-element to create the Gradient Border Glowing Animation around the .card
elements. I have set its height & width to 100%, and border width to “2px” but it’s colour to transparent.
css.card { --start: 0; position: relative; display: flex; flex-direction: column; justify-content: center; align-items: flex-start; width: 280px; height: 350px; margin: 10px; padding: 10px 40px; background-color: #040404; border-radius: 14px; transition: border-color 0.3s ease-in-out; } .card::before { position: absolute; content: ""; width: 100%; height: 100%; left: 50%; top: 50%; transform: translate(-50%, -50%); border-radius: 14px; border: 2px solid transparent; background: var(--gradient); background-attachment: fixed; mask: linear-gradient(#0000, #0000), conic-gradient(from calc(( var(--start) - (20 * 1.1) ) * 1deg), #ffffff1f 0deg, white, #ffffff00 100deg); mask-composite: intersect; mask-clip: padding-box, border-box; opacity: 0; transition: 0.5s ease; }
Then I specified .card::before
background
property to gradient by the --gradient
variable, setting the background-attachment property to fixed.
Then the main part comes, where I have used the mask property to define a combination of linear and conical gradients. By this masking technique, we have achieved a gradient effect that emanates from the border of the .card
elements.
cssmask: linear-gradient(#0000, #0000), conic-gradient(from calc(( var(--start) - (20 * 1.1) ) * 1deg), #ffffff1f 0deg, white, #ffffff00 100deg);
Then I set the mask-composite property to intersect, for a precise intersection of the two gradient layers. Additionally, I specified the mask-clip
property to padding-box & border-box.
css.card::before { mask-composite: intersect; mask-clip: padding-box, border-box; }
Also, I set the card’s Pseudo-element opacity to 0 by default and added a transition.
If you change its opacity: 0; to opacity: 1; then you will see the output like this,
Output of CSS Gradient Border
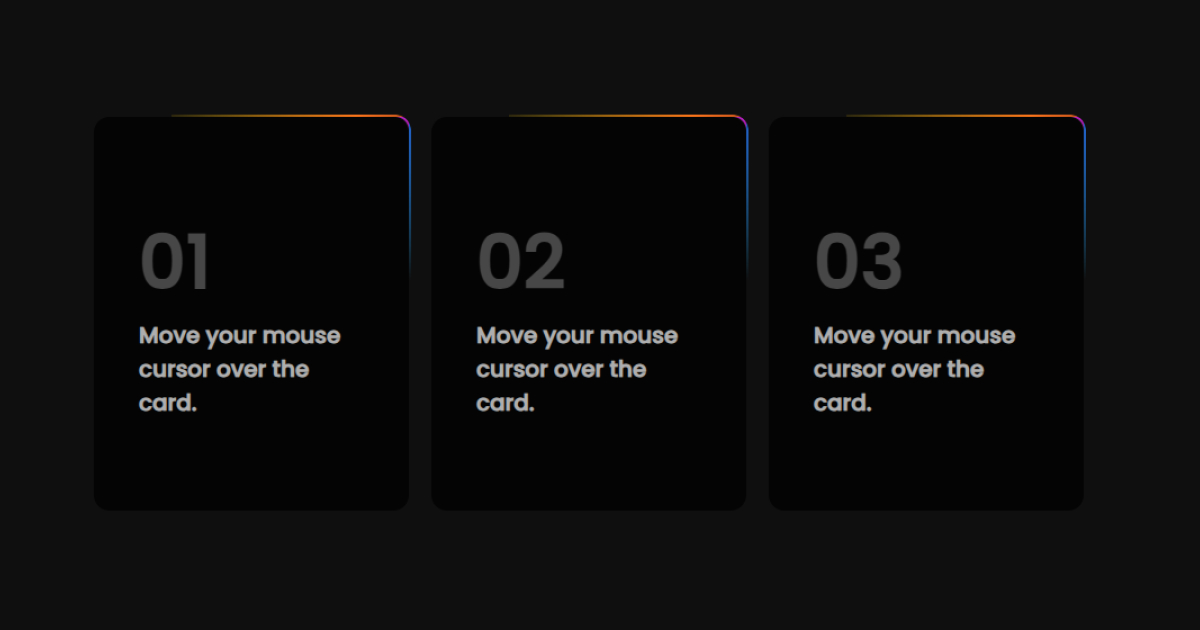
Add CSS Gradient Border Glow Effect
To add a glowing effect I have added some styles to.glow
class. First, I set its position to absolute, width, height, transform
property, etc.
The main part is I have set filter
property with a blur function, by which we can achieve a soft glow effect.
Additionally, styles added to its Pseudo-element .glow::before
are similar to the card’s Pseudo-element .card::before
css.glow { pointer-events: none; position: absolute; width: 100%; height: 100%; left: 50%; top: 50%; transform: translate(-50%, -50%); filter: blur(14px); } .glow::before { position: absolute; content: ""; width: 98%; height: 98%; left: 50%; top: 50%; transform: translate(-50%, -50%); border-radius: 14px; border: 15px solid transparent; background: var(--gradient); background-attachment: fixed; mask: linear-gradient(#0000, #0000), conic-gradient(from calc(( var(--start) - (20 * 1.1) ) * 1deg), #000 0deg, #ffffff, rgba(0, 0, 0, 0) 100deg); mask-composite: intersect; mask-clip: padding-box, border-box; opacity: 0; transition: 1s ease; } .card:hover > .glow::before { opacity: 1; } .card:hover::before { opacity: 0.6; }
.card:hover > .glow::before
it refers to when the .card
element is being hovered, the opacity of the .glow::before
will be ‘1’.
.card:hover::before
is for when the .card
elements are hovered over, the opacity of its ::before
pseudo-element will be ‘0.6’.
Adding animation functionality with JavaScript
We have so far designed the card gradient border glowing animation project using CSS.
Now it is time to enable the functionality using JavaScript that tracks the movement of the mouse cursor over the .card
elements and runs the animation accordingly by updating the --start
CSS variable.
So let’s break down the javascript code, First of all, I have created a variable called cards
, which stores all the .card
elements by using document.querySelectorAll()
method.
Then I have added an event listener for the mousemove
event on each .card
element using the forEach()
method and this will trigger the handleMouseMove
function. It means when the user moves the mouse over .card
elements handleMouseMove
function will be triggered.
javascriptconst cards = document.querySelectorAll(".card"); cards.forEach((card) => { card.addEventListener("mousemove", handleMouseMove); }); function handleMouseMove(e) { const rect = this.getBoundingClientRect(); const mouseX = e.clientX - rect.left - rect.width / 2; const mouseY = e.clientY - rect.top - rect.height / 2; let angle = Math.atan2(mouseY, mouseX) * (180 / Math.PI); angle = (angle + 360) % 360; this.style.setProperty("--start", angle + 60); }
In this handleMouseMove
function, I use getBoundingClientRect()
method, which returns an object with properties describing the element’s position and dimensions relative to the viewport.
javascriptconst mouseX = e.clientX - rect.left - rect.width / 2; const mouseY = e.clientY - rect.top - rect.height / 2;
Here I have created two variables mouseX
& mouseY
, these store the values by subtracting the element’s position and dimensions from the mouse coordinates, which precisely determines the position of the cursor within the boundaries of the element.
javascriptlet angle = Math.atan2(mouseY, mouseX) * (180 / Math.PI);
Then I use Math.atan2()
javascript method, which calculates the arctangent of the ratio between the mouseY
and mouseX
values and converted the value to degrees by multiplying it by (180 / Math.PI)
. I stored it in the angle
variable.
angle = (angle + 360) % 360;
this part is for the calculated angle to ensure it stays within a range of 0 to 360 degrees.
At last, using the CSSStyleDeclaration.setProperty()
method dynamically updates the value of the CSS custom property named --start
by utilizing the calculated value of the angle
variable.
javascriptthis.style.setProperty("--start", angle + 60);
Note that I added 60 degrees to the calculated angle
variable to adjust the initial position of the CSS gradient border.
Output of CSS Gradient Border Glowing Animation Hover Effect - Live Experience
See the Pen CSS Gradient Border Glowing Animation Hover Effect on CodePen.
So this tutorial was about How to create a Gradient Border Glowing Animation Hover Effect using HTML, CSS, and JavaScript. Throughout the tutorial, you have learned about CSS Gradient Border. I tried to explain to you the step-by-step process of building the Gradient Border Glowing Animation Hover Effect using HTML, CSS, and JavaScript. I hope this was very helpful to you.
Read also: Create a Dropdown Select Menu in ReactJs